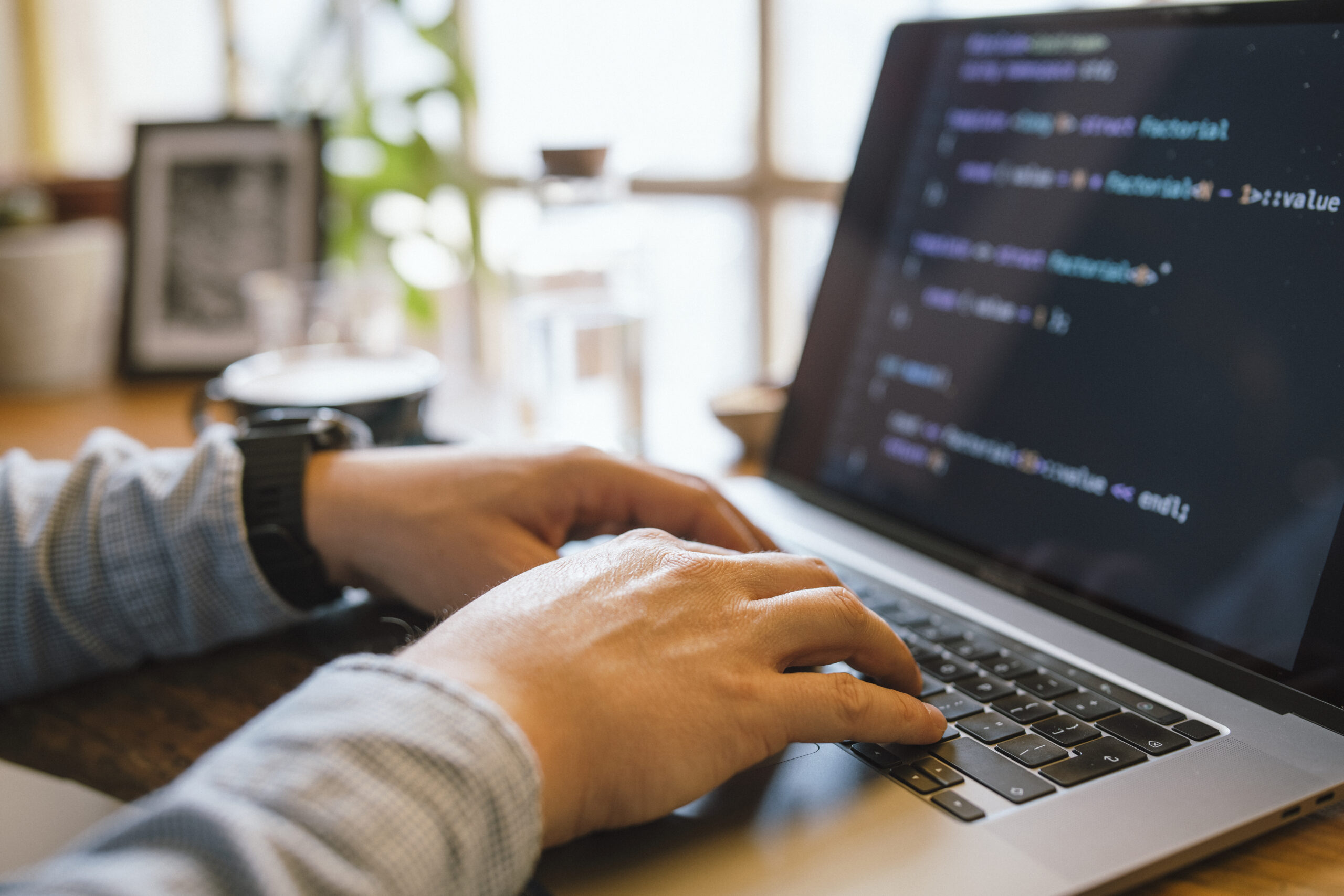
Debugging is one of the most crucial — still normally ignored — expertise in the developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why items go Completely wrong, and Discovering to think methodically to solve issues efficiently. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can preserve hours of aggravation and significantly boost your productivity. Listed here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the fastest means builders can elevate their debugging capabilities is by mastering the equipment they use daily. Whilst writing code is a person Component of growth, realizing how you can interact with it proficiently for the duration of execution is equally vital. Modern-day advancement environments come Geared up with potent debugging abilities — but numerous builders only scratch the floor of what these resources can perform.
Just take, by way of example, an Integrated Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools permit you to set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in many cases modify code about the fly. When employed correctly, they Enable you to observe just how your code behaves throughout execution, which happens to be invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe network requests, perspective actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage about running processes and memory administration. Discovering these resources could possibly have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Variation control techniques like Git to be aware of code historical past, find the exact second bugs ended up launched, and isolate problematic variations.
In the end, mastering your equipment suggests likely past default options and shortcuts — it’s about establishing an personal expertise in your improvement setting to make sure that when problems occur, you’re not shed in the dark. The better you understand your equipment, the greater time you are able to commit fixing the particular challenge in lieu of fumbling as a result of the procedure.
Reproduce the condition
One of the most critical — and infrequently overlooked — steps in helpful debugging is reproducing the condition. Right before leaping to the code or producing guesses, developers need to produce a reliable setting or situation exactly where the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a recreation of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Inquire thoughts like: What steps brought about the issue? Which ecosystem was it in — progress, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have, the much easier it turns into to isolate the exact circumstances less than which the bug happens.
When you’ve gathered plenty of data, attempt to recreate the problem in your neighborhood environment. This might imply inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the edge scenarios or state transitions included. These checks not just support expose the problem and also prevent regressions Later on.
In some cases, The problem can be environment-precise — it might come about only on sure operating programs, browsers, or underneath particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the problem isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, as well as a methodical technique. But as you can consistently recreate the bug, you're presently halfway to repairing it. By using a reproducible state of affairs, You may use your debugging resources a lot more effectively, check prospective fixes safely and securely, and talk far more Plainly with the staff or people. It turns an summary complaint into a concrete obstacle — Which’s where by builders prosper.
Read through and Recognize the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders really should study to deal with error messages as direct communications from the procedure. They normally show you what exactly occurred, exactly where it transpired, and in some cases even why it took place — if you know how to interpret them.
Start by examining the concept very carefully and in whole. A lot of developers, specially when beneath time stress, look at the 1st line and right away begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just duplicate and paste error messages into search engines like yahoo — study and have an understanding of them 1st.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line number? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also handy to know the terminology of your programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java often observe predictable patterns, and Discovering to acknowledge these can dramatically increase your debugging procedure.
Some glitches are vague or generic, and in Those people scenarios, it’s vital to look at the context by which the mistake occurred. Test associated log entries, input values, and up to date improvements inside the codebase.
Don’t ignore compiler or linter warnings both. These normally precede more substantial challenges and provide hints about possible bugs.
Eventually, mistake messages are not your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, helping you pinpoint concerns more rapidly, lower debugging time, and turn into a more effective and assured developer.
Use Logging Properly
Logging is The most impressive tools in the developer’s debugging toolkit. When made use of effectively, it provides genuine-time insights into how an application behaves, aiding you recognize what’s going on under the hood without needing to pause execution or stage with the code line by line.
An excellent logging approach begins with realizing what to log and at what degree. Frequent logging ranges contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data for the duration of advancement, Information for basic occasions (like successful start-ups), Alert for probable troubles that don’t break the applying, Mistake for genuine troubles, and FATAL when the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure critical messages and slow down your technique. Give attention to key situations, condition changes, enter/output values, and demanding conclusion factors inside your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without having halting This system. They’re Specifically important in creation environments where by stepping by way of code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Using a very well-thought-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your applications, and Enhance the Over-all maintainability and reliability of the code.
Assume Like a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To efficiently establish and fix bugs, developers have to solution the process like a detective fixing a thriller. This way of thinking allows stop working complex concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the trouble: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, obtain just as much applicable information and facts as you can without jumping to conclusions. Use logs, check circumstances, and person stories to piece alongside one another a transparent photo of what’s occurring.
Up coming, sort hypotheses. Check with by yourself: What might be causing this behavior? Have any variations recently been built into the codebase? Has this difficulty transpired ahead of beneath equivalent situations? The goal is to slender down opportunities and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in a very controlled surroundings. In the event you suspect a specific functionality or part, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Enable the outcome lead you nearer to the reality.
Shell out close consideration to little aspects. Bugs generally conceal in the the very least anticipated places—similar to a missing semicolon, an off-by-just one error, or simply a race issue. Be thorough and affected individual, resisting the urge to patch The problem with out thoroughly knowing it. Temporary fixes may possibly hide the true problem, only for it to resurface afterwards.
And finally, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging method can help save time for long term difficulties and aid Many others realize your reasoning.
By wondering like a detective, builders can sharpen their analytical abilities, technique problems methodically, and develop into more practical at uncovering hidden troubles in elaborate techniques.
Generate Tests
Creating assessments is among the simplest methods to boost your debugging techniques and overall improvement efficiency. Tests not merely enable capture bugs early but also serve as a safety net that gives website you self-assurance when building variations to your codebase. A well-tested application is easier to debug since it permits you to pinpoint just the place and when a challenge happens.
Begin with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can promptly expose whether a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you promptly know wherever to glance, appreciably cutting down enough time put in debugging. Unit tests are Primarily handy for catching regression bugs—difficulties that reappear soon after Formerly becoming fixed.
Future, combine integration exams and finish-to-end checks into your workflow. These support make certain that numerous parts of your software perform together effortlessly. They’re specially beneficial for catching bugs that happen in elaborate programs with several components or expert services interacting. If anything breaks, your tests can show you which Component of the pipeline failed and less than what problems.
Creating checks also forces you to definitely Consider critically about your code. To check a function thoroughly, you may need to know its inputs, predicted outputs, and edge instances. This standard of knowing naturally qualified prospects to better code construction and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. When the test fails persistently, you could target correcting the bug and observe your take a look at pass when The problem is solved. This approach makes sure that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a annoying guessing activity into a structured and predictable method—serving to you capture more bugs, a lot quicker and more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s straightforward to be immersed in the condition—looking at your display screen for several hours, seeking Answer right after Resolution. But One of the more underrated debugging tools is just stepping away. Using breaks can help you reset your intellect, cut down frustration, and often see the issue from a new perspective.
When you're too close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind results in being fewer successful at challenge-fixing. A short walk, a espresso crack, or maybe switching to a unique process for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support protect against burnout, In particular in the course of lengthier debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but additionally draining. Stepping away helps you to return with renewed Electricity as well as a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you prior to.
For those who’re caught, a good general guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all over, stretch, or do a thing unrelated to code. It might sense counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise strategy. It provides your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of resolving it.
Discover From Every single Bug
Each individual bug you experience is much more than simply A short lived setback—It is a chance to improve as a developer. No matter whether it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a little something valuable should you make the effort to replicate and analyze what went Erroneous.
Get started by inquiring yourself several essential issues as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit screening, code evaluations, or logging? The solutions typically reveal blind spots within your workflow or comprehension and make it easier to Make much better coding behaviors transferring ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you acquired. Eventually, you’ll begin to see designs—recurring concerns or frequent errors—that you could proactively avoid.
In team environments, sharing Anything you've acquired from the bug along with your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short create-up, or A fast expertise-sharing session, aiding others steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are not the ones who generate excellent code, but those who continually learn from their problems.
In the end, Every single bug you fix adds a completely new layer for your ability established. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.